I recently completed a LinkedIn course on Java Lambdas and Streams, and it has been an eye-opening journey into writing more expressive, functional, and efficient Java code. Lambdas and Streams, introduced in Java 8, have revolutionized the way we write and process collections, making code more concise and readable.
Why Use Java Lambdas?
Lambdas provide a functional approach to writing Java code, eliminating boilerplate and making anonymous functions more intuitive. Instead of writing bulky anonymous classes, we can use a more streamlined syntax:
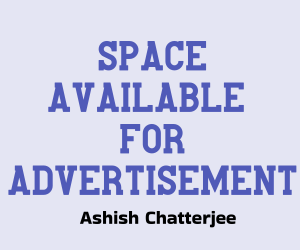
Traditional Approach:
javaCopyEditComparator<String> comparator = new Comparator<String>() {
@Override
public int compare(String s1, String s2) {
return s1.length() - s2.length();
}
};
Lambda Approach:
javaCopyEditComparator<String> comparator = (s1, s2) -> s1.length() - s2.length();
This not only reduces code but also improves clarity.
Unlocking the Power of Streams
The Streams API allows for efficient data processing by enabling functional-style operations on collections. Instead of writing loops, we can chain powerful methods like filter()
, map()
, and reduce()
.
Example:
javaCopyEditList<String> names = Arrays.asList("Alice", "Bob", "Anna", "Steve");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
System.out.println(filteredNames); // Output: [Alice, Anna]
Streams leverage lazy evaluation, improving performance when working with large datasets.
Final Thoughts
Mastering Lambdas and Streams is essential for writing modern Java applications. They enhance productivity, improve code readability, and optimize performance. I highly recommend every Java developer to explore these concepts further.
Here’s my LinkedIn certificate, marking another step in my Java learning journey! 🚀 #Java #Lambdas #Streams #Learning #Programming
Github repo link:
GitHub – AshishChatterjee/JavaLambdaStream
Video on the above topic will be available soon :
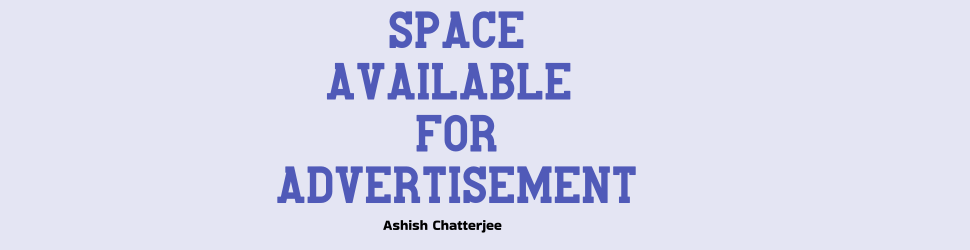
GIPHY App Key not set. Please check settings