Introduction
Face recognition is a biometric technology that identifies or verifies individuals based on their facial features. It is widely used in security, authentication, surveillance, and personalization applications. This blog delves into the concept of face recognition, different algorithms used, and provides implementation examples in Python and Java.
What is Face Recognition?
Face recognition is an advanced computer vision technology that identifies human faces in images or videos and matches them against stored records. It works in multiple stages:
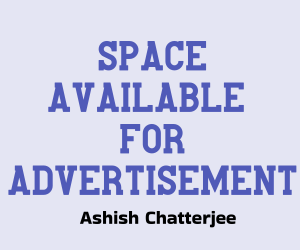
- Face Detection – Identifies and locates faces in an image.
- Feature Extraction – Analyzes unique facial landmarks.
- Face Matching – Compares the extracted features with stored templates to verify identity.
Applications of Face Recognition
- Security & Surveillance – Law enforcement uses facial recognition to track criminals.
- Authentication – Mobile devices and secure access systems use it for identity verification.
- Retail & Marketing – Personalized advertisements based on customer demographics.
- Healthcare – Patient verification and monitoring.
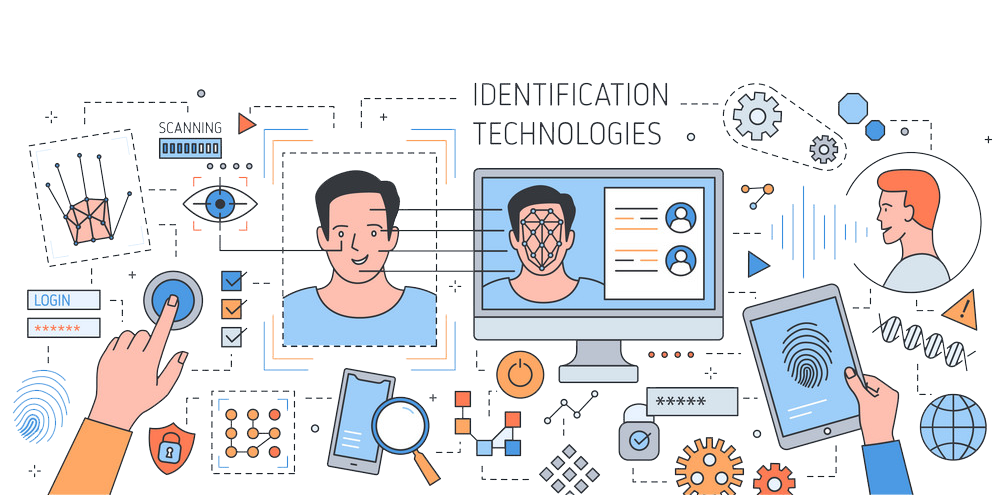
Types of Face Recognition Algorithms
1. Knowledge-Based (Rule-Based) Methods
These methods use predefined rules about facial features such as eye distance, nose position, and mouth shape to detect faces. However, they struggle with variations in facial orientation, lighting, and occlusion.
2. Feature-Based Methods
These techniques analyze facial features such as edges, textures, and geometric relationships. Examples include:
- Histogram of Oriented Gradients (HOG) – Extracts object features based on gradient orientations.
- Viola-Jones Algorithm – Uses Haar-like features and AdaBoost classifier for real-time face detection.
3. Template Matching Methods
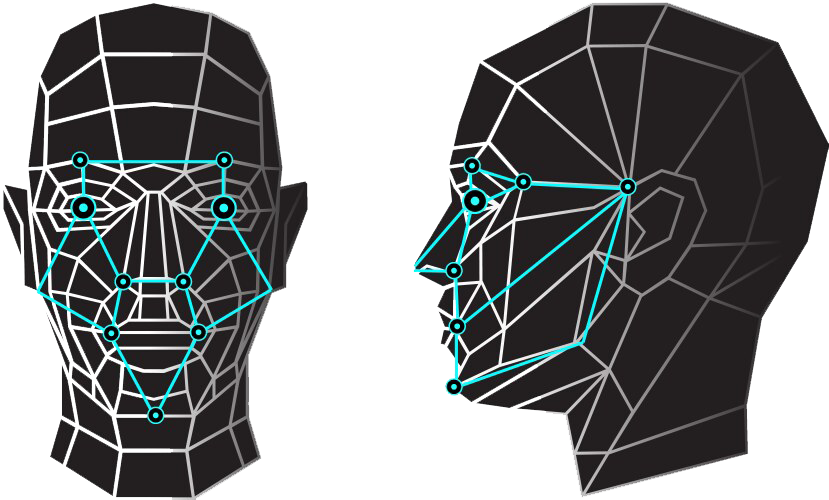
These methods compare an input image with stored facial templates.
- Eigenfaces and Fisherfaces – Utilize Principal Component Analysis (PCA) and Linear Discriminant Analysis (LDA) to recognize faces.
- Local Binary Patterns Histogram (LBPH) – Extracts texture-based facial features for robust recognition.
4. Appearance-Based Methods (Statistical and Machine Learning)
These algorithms use statistical models and machine learning techniques to learn facial patterns.
- Support Vector Machines (SVMs) – Classifies faces based on feature vectors.
- Neural Networks (MLP, CNNs) – Deep learning-based models like FaceNet, DeepFace, and OpenFace use Convolutional Neural Networks (CNNs) to extract deep facial features.
5. Deep Learning-Based Face Recognition
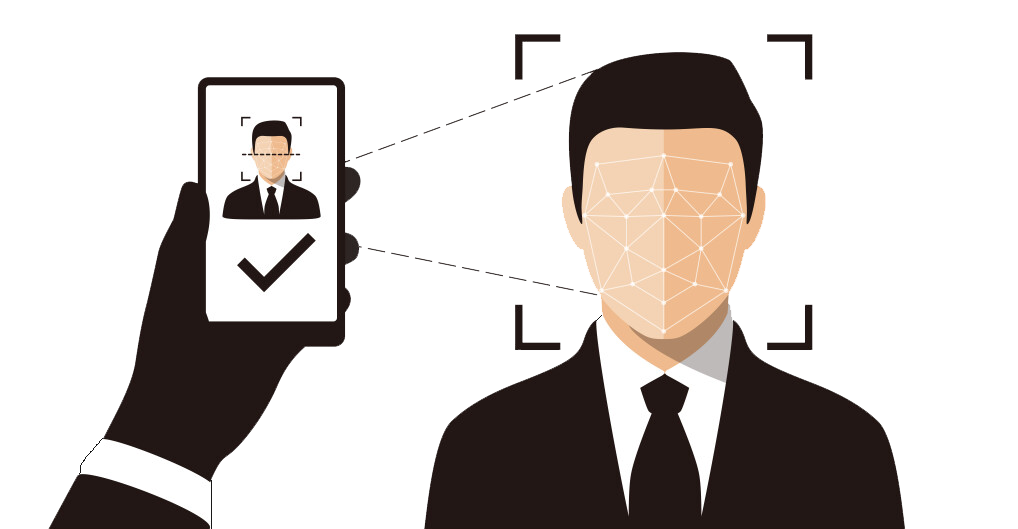
Modern face recognition systems leverage deep learning models to achieve high accuracy.
- FaceNet (Google’s Model) – Uses a deep CNN with triplet loss for high-accuracy recognition.
- DeepFace (Facebook’s Model) – Uses deep neural networks for real-time recognition.
- Dlib Face Recognition – Uses deep metric learning to generate 128D facial embeddings.
6. Hybrid Methods
Combining multiple approaches can improve recognition accuracy and robustness.
- CNN + HOG – Extracts deep facial features while using HOG for faster processing.
- LBPH + SVM – Uses LBPH for feature extraction and SVM for classification.
- FaceNet + Triplet Loss – Learns a facial embedding space for superior face matching.
Face Recognition Implementation
Python Implementation Using OpenCV and Dlib
Python provides powerful libraries such as OpenCV and Dlib for face recognition.
Installing Dependencies
pip install opencv-python dlib face_recognition numpy
Python Code for Face Recognition
import cv2
import face_recognition
# Load an image and encode it
known_image = face_recognition.load_image_file("known_face.jpg")
known_encoding = face_recognition.face_encodings(known_image)[0]
# Load an unknown image
unknown_image = face_recognition.load_image_file("unknown_face.jpg")
unknown_encoding = face_recognition.face_encodings(unknown_image)[0]
# Compare faces
results = face_recognition.compare_faces([known_encoding], unknown_encoding)
print("Face Match: ", results[0])
Java Implementation Using OpenCV
Java developers can use OpenCV to implement face recognition.
Setting Up OpenCV in Java
- Download OpenCV and add
opencv-XXX.jar
to the project. - Load the native OpenCV library.
Java Code for Face Detection
import org.opencv.core.*;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.objdetect.CascadeClassifier;
import org.opencv.imgproc.Imgproc;
public class FaceDetection {
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args) {
CascadeClassifier faceDetector = new CascadeClassifier("haarcascade_frontalface_default.xml");
Mat image = Imgcodecs.imread("input.jpg");
MatOfRect faces = new MatOfRect();
faceDetector.detectMultiScale(image, faces);
for (Rect rect : faces.toArray()) {
Imgproc.rectangle(image, new Point(rect.x, rect.y),
new Point(rect.x + rect.width, rect.y + rect.height),
new Scalar(0, 255, 0));
}
Imgcodecs.imwrite("output.jpg", image);
}
}
There are several types of Face Recognition Algorithms, categorized based on their approach and underlying techniques. Below are the major types:
1. Knowledge-Based (Rule-Based) Algorithms
These algorithms rely on predefined rules about facial features such as eye distance, nose position, and mouth shape to detect and recognize faces.
Example:
- Rule-Based Face Detection – Uses manually crafted rules to detect face structures.
Pros:
✅ Simple and intuitive approach.
✅ Works well in constrained environments.
Cons:
❌ Struggles with variations in lighting, pose, and occlusions.
❌ Not scalable for complex real-world applications.
2. Feature-Based Methods
These techniques extract distinctive facial features such as edges, corners, textures, and skin color, and then match them with stored facial templates.
Popular Algorithms:
- Histogram of Oriented Gradients (HOG) – Extracts gradient-based features from an image for recognition.
- Viola-Jones Algorithm – Uses Haar-like features and AdaBoost for fast face detection.
- Active Shape Models (ASM) & Active Appearance Models (AAM) – Model facial variations for recognition.
Pros:
✅ Computationally efficient.
✅ Works in real-time applications.
Cons:
❌ Sensitive to variations in lighting and expressions.
❌ May require additional pre-processing for accuracy.
3. Template Matching Methods
These algorithms compare an input face image with pre-stored facial templates.
Popular Algorithms:
- Eigenfaces (PCA-based method) – Uses Principal Component Analysis (PCA) to reduce dimensionality and match face features.
- Fisherfaces (LDA-based method) – Uses Linear Discriminant Analysis (LDA) to improve recognition under varying lighting conditions.
- Local Binary Patterns Histogram (LBPH) – Extracts texture-based local features for robust recognition.
Pros:
✅ Simple and effective in controlled environments.
✅ LBPH works well with grayscale images and low-resolution images.
Cons:
❌ PCA and LDA struggle with variations in expressions and poses.
❌ Requires a well-maintained face template database.
4. Appearance-Based Methods (Statistical and Machine Learning)
These algorithms use statistical models and machine learning techniques to learn facial patterns.
Popular Algorithms:
- Support Vector Machines (SVMs) – Classifies faces based on feature vectors.
- Neural Networks (MLP, CNNs) – Deep learning-based models like FaceNet, DeepFace, and OpenFace use Convolutional Neural Networks (CNNs) to extract deep facial features.
Pros:
✅ More accurate than rule-based methods.
✅ Works well with large datasets.
Cons:
❌ Requires large amounts of training data.
❌ Computationally expensive.
5. Deep Learning-Based Face Recognition
Modern face recognition systems leverage deep learning models to achieve high accuracy.
Popular Algorithms:
- FaceNet (Google’s Model) – Uses a deep CNN with triplet loss for high-accuracy recognition.
- DeepFace (Facebook’s Model) – Uses deep neural networks for real-time recognition.
- Dlib Face Recognition – Uses deep metric learning to generate 128D facial embeddings.
Pros:
✅ Highly accurate, even under variations in lighting, pose, and occlusions.
✅ Self-learning and adaptable to new faces.
Cons:
❌ Requires significant computational power (GPUs).
❌ Needs large datasets for training.
6. Hybrid Methods
Combining multiple approaches can improve recognition accuracy and robustness.
Examples:
- CNN + HOG – Extracts deep facial features while using HOG for faster processing.
- LBPH + SVM – Uses LBPH for feature extraction and SVM for classification.
- FaceNet + Triplet Loss – Learns a facial embedding space for superior face matching.
Pros:
✅ Achieves high accuracy.
✅ More robust against variations in the environment.
Cons:
❌ Computationally intensive.
❌ Requires a well-optimized model.
Conclusion
Different face recognition algorithms suit different use cases. Traditional methods like PCA, LDA, and LBPH are useful for lightweight applications, while deep learning models like FaceNet and DeepFace provide superior accuracy and robustness.
Face recognition is a powerful AI-driven technology used in numerous applications. From traditional rule-based methods to deep learning approaches, the field has evolved significantly. Python and Java provide efficient libraries to implement face recognition in real-world projects.
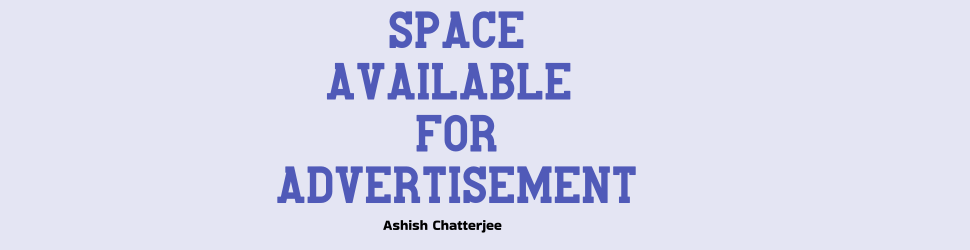
GIPHY App Key not set. Please check settings