Django, a high-level Python web framework, follows the Model-View-Template (MVT) architectural pattern, which is a variant of the popular Model-View-Controller (MVC) architecture. MVT helps developers create robust and scalable web applications efficiently by maintaining a clear separation of concerns.
What is MVT Architecture?
MVT stands for:
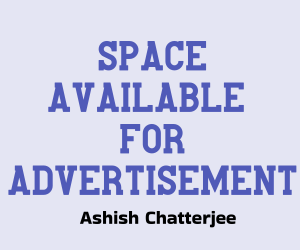
- Model – Handles the database and business logic.
- View – Manages the user interface and application logic.
- Template – Defines the presentation layer (HTML + dynamic content).
Unlike traditional MVC frameworks, where the Controller handles user requests and updates the model, Django’s MVT framework manages requests internally, reducing the need for explicit controller code.
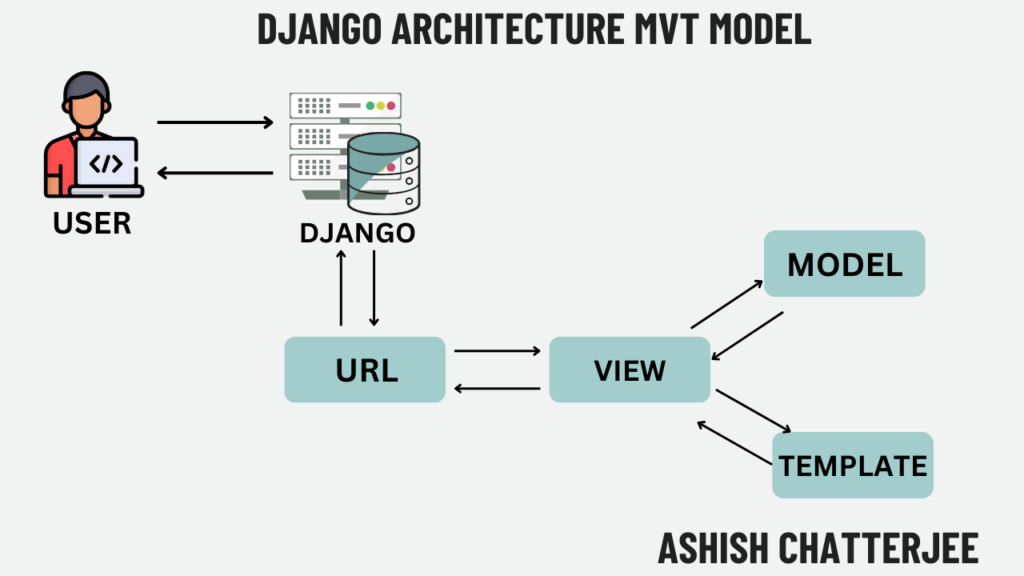
Components of MVT
1. Model
The Model represents the data structure and interacts with the database. It defines the schema, constraints, and relationships between data objects.
Example:
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=255)
price = models.DecimalField(max_digits=10, decimal_places=2)
description = models.TextField()
def __str__(self):
return self.name
This model defines a Product
table with fields for name, price, and description.
2. View
The View processes HTTP requests, fetches data from the model, and renders the appropriate template. Views act as the bridge between models and templates.
Example:
from django.shortcuts import render
from .models import Product
def product_list(request):
products = Product.objects.all()
return render(request, 'product_list.html', {'products': products})
This view fetches all products from the database and passes them to the product_list.html
template.
3. Template
Templates control the presentation layer, combining static HTML with dynamic data using Django’s template language.
Example:
<!DOCTYPE html>
<html>
<head>
<title>Product List</title>
</head>
<body>
<h1>Products</h1>
<ul>
{% for product in products %}
<li>{{ product.name }} - ${{ product.price }}</li>
{% endfor %}
</ul>
</body>
</html>
This template dynamically displays product names and prices.
How MVT Works in Django
- User Request: The user accesses a URL in the browser.
- URL Dispatcher: Django routes the request to the appropriate view based on URL patterns.
- View Logic: The view retrieves data from the model and renders it using a template.
- Response: The template generates an HTML response, which is sent to the user’s browser.
Example URL Configuration:
from django.urls import path
from .views import product_list
urlpatterns = [
path('products/', product_list, name='product_list'),
]
This routes the /products/
URL to the product_list
view.
Advantages of MVT in Django
- Separation of Concerns: Clear distinction between logic (Model), presentation (Template), and control (View).
- Rapid Development: Built-in ORM and templating engine streamline development.
- Scalability: Suitable for small to large-scale applications.
- Code Reusability: Reusable components improve maintainability.
Conclusion
Django’s MVT architecture simplifies web development by providing a structured way to manage data, business logic, and user interfaces. Understanding MVT is essential for building efficient and scalable Django applications.
If you’re new to Django, try implementing a simple project using MVT to get hands-on experience!
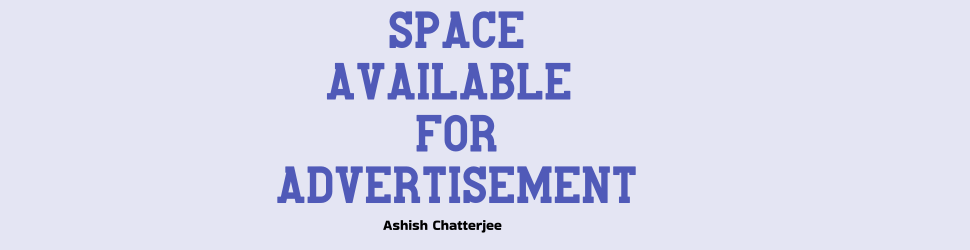
GIPHY App Key not set. Please check settings