Introduction
Software engineering has evolved to emphasize maintainability, scalability, and modularity in application development. The S.O.L.I.D principles, introduced by Robert C. Martin, are fundamental object-oriented design principles that promote high-quality, adaptable, and robust software architectures. Each principle addresses specific design challenges to ensure better system organization and long-term sustainability.
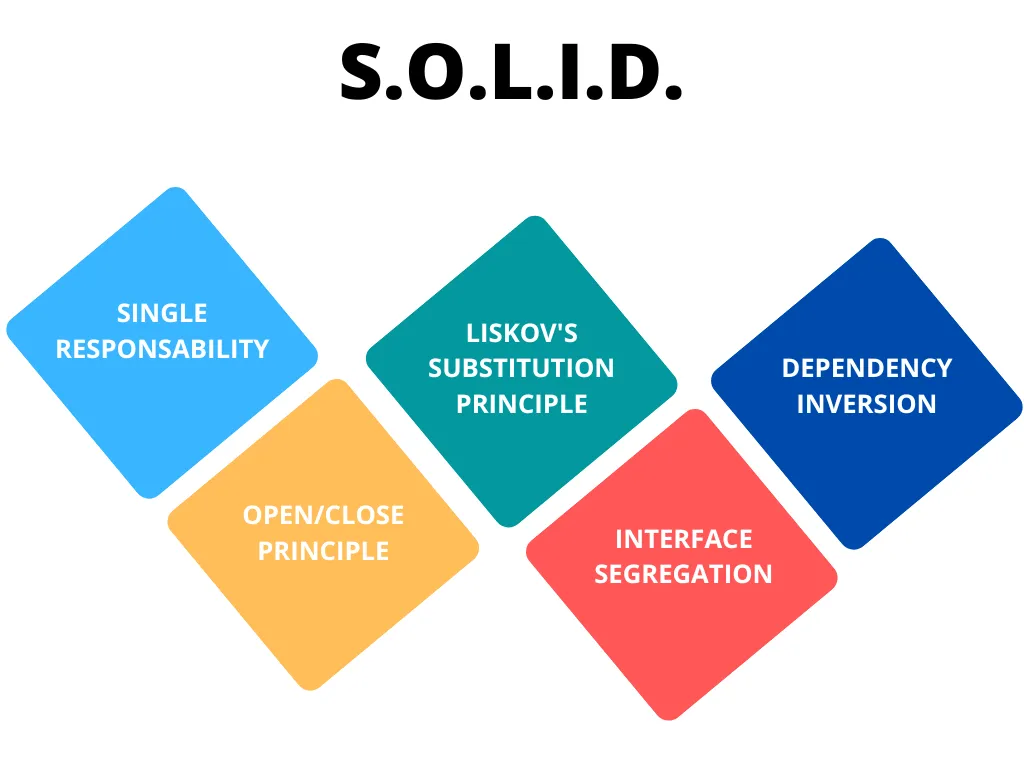
1. Single Responsibility Principle (SRP)
Definition
The Single Responsibility Principle states that a class should have only one reason to change, meaning it should be responsible for only one aspect of the software’s functionality.
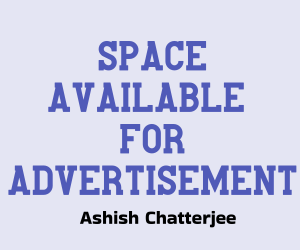
Analysis
- Encourages modular design by ensuring that each class has a well-defined purpose.
- Enhances maintainability and readability by preventing bloated, monolithic classes.
- Reduces the risk of unintentional side effects when modifying code.
Real-World Example
Consider a ReportManager
class responsible for fetching data, formatting reports, and exporting them. Violating SRP, the class becomes difficult to manage as responsibilities grow. A better design is to split it into:
ReportFetcher
: Handles data retrieval.ReportFormatter
: Formats data into a structured report.ReportExporter
: Exports reports in different formats.
This modular approach makes maintenance easier and reduces interdependencies.
Java Example
class ReportFetcher {
public String fetchData() {
return "Report Data";
}
}
class ReportFormatter {
public String format(String data) {
return "Formatted: " + data;
}
}
class ReportExporter {
public void export(String formattedData) {
System.out.println("Exporting: " + formattedData);
}
}
Benefits and Challenges
Benefits:
- Improved code organization and maintainability.
- Easier debugging and testing since responsibilities are isolated.
- Increased reusability across different modules.
Challenges:
- Requires careful design planning.
- May result in many smaller classes, which could be challenging for beginners.
2. Open-Closed Principle (OCP)
Definition
Software entities (classes, modules, functions) should be open for extension but closed for modification.
Analysis
- Allows extending functionality without modifying existing code.
- Reduces the risk of introducing bugs in stable code.
- Encourages the use of polymorphism and abstraction.
Real-World Example
A PaymentProcessor
class directly handling multiple payment methods violates OCP. Instead, using an abstract PaymentMethod
class with subclasses (CreditCardPayment
, PayPalPayment
) allows adding new payment options without altering the PaymentProcessor
class.
Java Example
interface PaymentMethod {
void pay(double amount);
}
class CreditCardPayment implements PaymentMethod {
public void pay(double amount) {
System.out.println("Paid " + amount + " using Credit Card.");
}
}
class PayPalPayment implements PaymentMethod {
public void pay(double amount) {
System.out.println("Paid " + amount + " using PayPal.");
}
}
class PaymentProcessor {
public void processPayment(PaymentMethod method, double amount) {
method.pay(amount);
}
}
3. Liskov Substitution Principle (LSP)
Definition
Subtypes must be substitutable for their base types without altering program correctness.
Real-World Example
Consider a Rectangle
class with width
and height
attributes. A Square
subclass overriding setWidth()
and setHeight()
disrupts expected behavior since setting width affects height as well. This violates LSP.
Java Example
class Rectangle {
protected int width, height;
public void setWidth(int width) { this.width = width; }
public void setHeight(int height) { this.height = height; }
public int getArea() { return width * height; }
}
class Square extends Rectangle {
public void setWidth(int width) {
this.width = this.height = width;
}
public void setHeight(int height) {
this.width = this.height = height;
}
}
4. Interface Segregation Principle (ISP)
Definition
Clients should not be forced to depend on interfaces they do not use.
Java Example
interface Printer {
void print();
}
interface Scanner {
void scan();
}
class BasicPrinter implements Printer {
public void print() {
System.out.println("Printing document...");
}
}
class MultiFunctionPrinter implements Printer, Scanner {
public void print() {
System.out.println("Printing document...");
}
public void scan() {
System.out.println("Scanning document...");
}
}
5. Dependency Inversion Principle (DIP)
Definition
High-level modules should not depend on low-level modules. Both should depend on abstractions.
Java Example
interface NotificationSender {
void sendNotification(String message);
}
class EmailSender implements NotificationSender {
public void sendNotification(String message) {
System.out.println("Email sent: " + message);
}
}
class SMSNotifier implements NotificationSender {
public void sendNotification(String message) {
System.out.println("SMS sent: " + message);
}
}
class NotificationService {
private NotificationSender sender;
public NotificationService(NotificationSender sender) {
this.sender = sender;
}
public void notifyUser(String message) {
sender.sendNotification(message);
}
}
Conclusion
The S.O.L.I.D principles are crucial for designing maintainable, scalable, and flexible software systems. By applying these principles, developers can create modular architectures that accommodate changes with minimal disruption. Understanding and implementing these principles enhances code reusability, reduces technical debt, and improves system robustness.
References
- GeeksforGeeks: S.O.L.I.D Principles
- freeCodeCamp: S.O.L.I.D Explained
- DigitalOcean: S.O.L.I.D Principles
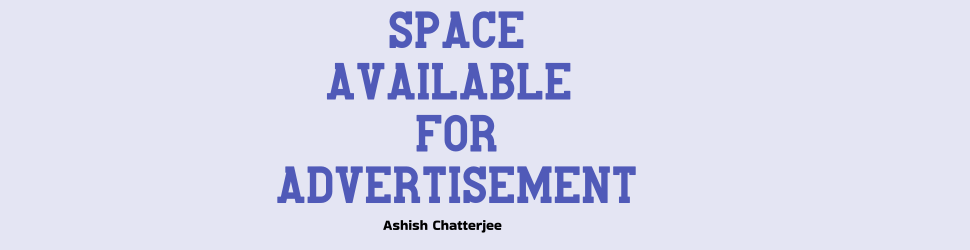
GIPHY App Key not set. Please check settings